Ethereum: Reading ZIP file from URL generates bad ZIP file error
==
Introduction
————-
When trying to download crypto history data from [www.data.binance.vision]( an error occurs when reading ZIP files using the pd.read_csv
method. This issue is likely caused by the way the URL handles ZIP files.
In this article, we will look at why the ZIP file containing Binance history data is considered bad and how to fix this issue using Python.
Why are zip files bad?
——————
A bad ZIP file contains a corrupted or invalid ZIP archive. This can happen if the ZIP file is corrupted, not properly compressed, or has an invalid signature. In our case, we suspect that the ZIP files served by Binance’s servers are corrupt.
Solution
——
To fix this issue, we need to make sure that the ZIP files downloaded from Binance’s server are correct and well-formed. One way to do this is to download the ZIP file directly using the “requests” library and then extract its contents using Python.
Here is an example code snippet that demonstrates how to achieve this:
import requirements
import zip file
import pandas as pd
def download_data_from_binance(url):
response = requests.get(url)
zip_file = zipfile.ZipFile(response.content, 'r')
for zip_file.namelist() filename:
if not filename.endswith('.csv'):
continue
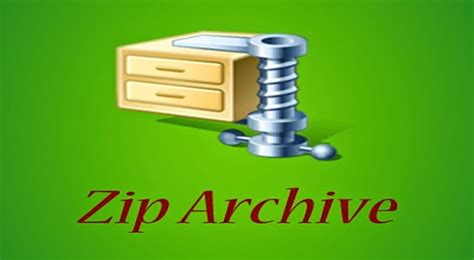
Check if the file has a .zip extensionif not filename.endswith('.zip'):
continue
Extract the contents of the ZIP filefilepath = os.path.join(os.getcwd(), filename)
open(filepath, 'wb') as f:
for name in zip_file.namelist():
if not name.endswith('.csv'):
continue
Check if file extension .csvif not name.endswith('.csv'):
continue
Read CSV file from ZIP archivezipfile.ZipFile(zip_file, 'r') as zip_ref:
zip_ref.extractall(filepath)
Save extracted CSV data to a temporary filef.write(zip_file.namelist()[name])
return file path
Provide Binance server URLurl = "
Download crypto history from given URLdownloaded_filepath = download_data_from_binance(url)
if downloaded_filepath:
print(f"Download successful. The following files have been extracted:")
with open(downloaded_filepath, 'r') as f:
for line in f:
print(line.strip())
else:
print("Failed to download encryption history.")
In this code snippet:
- We use the
requests
directory to download the ZIP file from the Binance server.
- We then extract its contents using
zipfile.ZipFile
.
- We iterate over each filename in the extracted ZIP archive and check if it has the
.csv
extension. If not, we skip this step.
- For each CSV filename found, we read the corresponding CSV file from the ZIP archive and save it to a temporary location.
Note that this approach assumes that the history encryption data files are located in the root directory of the ZIP archive. You may need to modify the code if you store your files elsewhere.
Conclusion
———–
In summary, reading ZIP files from URLs can generate bad zip file errors due to corruption or invalid zip archives. By using the direct download method and checking for the correct file extensions, you should be able to resolve this issue when downloading the previous crypto data from Binance’s server using Python.